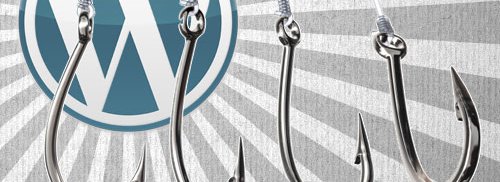
Basic Knowledge of Hooks in Your WordPress Themes
All in one word, hooks are built into WordPress or wordpress themes and are used to modify or add functionality to the core system without modifying core WordPress files at all.
The Requirement of Hooks
Let’s assume for a moment that WordPress does not provide any hooks. And let’s also say you work with a lot of scheduled posts and you would like to email yourself when a post is published. How would you go about doing that?
Without hooks you would need to modify core files. You would hunt down the code that is responsible for publishing a post and just after the function that performs the action, you would paste your own code.
This is detrimental for a number of reasons. The two big ones are updates and uncontrollable code. If you modify core WordPress files your code will work just fine but will be erased when you update WordPress Themes to the next version. You would have to remember or track all of your changes and then put them back in. Not exactly convenient. Alternatively, you could simply not update WordPress, but that would be a huge security risk in the long run.
Even if you do manage to keep track of changes and update WordPress Themes, you are left with a non-standard environment with uncontrollable code. Other WordPress developers will have a hard time dealing with your code, and the community as a whole will not be happy.
Enter Hooks
A hook does almost exactly what we talked about before (find the place where a post is published, paste your code), but replaces the horrible pasting bit with a placeholder. By default, there is a placeholder after the post publishing function, which allows you to tie functionality there. Here’s a shortened version of the actual wp_publish_post(), which you can find in wp-includes/post.php.
1 | function wp_publish_post( $post ) { // Stuff that actually published the post do_action( 'wp_insert_post', $post->ID, $post, true ); } |
That do_action()
bit basically says: You can tie your own function to me, they will all be executed. So whenever WordPress publishes a post using the wp_publish_post()
function it goes through all the necessary steps to make it happen. It then moves on to the do_action()
function. It’s first parameter is wp_insert_post
. Any function which is tied to this wp_insert_post
action will get executed.
Let’s look at an example.
To create a hooked function you need to create a simple plugin, use your theme’sfunctions.php file, or create a child theme and use the functions.php file in there. Let’s create a quick plugin now.
Create a new folder in the Plugins directory and name it “hook-example.” Create a “hook-example.php” file within that directory and paste the following code into it:
1 | <?php /** * Plugin Name: Hook Test * Description: A simple plugin to test hooks */ |
Your plugin is now ready. Head on over to the Plugins section in WordPress admin and activate it. Now let’s create a hooked function. In the hook-example.php
file, paste the following:
1 | add_action( 'wp_insert_post' , 'email_post_author' , 10, 3 ); function email_post_author( $post_id , $post , $update ) { $email = 'mymail@mail.com' ; $subject = 'New Post Published' ; $message = 'A new post was published, use this link to view it: ' . get_permalink( $post ->ID ); wp_mail( $email , $subject , $message ); } |
We’re using the add_action()
function to let WordPress know that we’d like to hook a function into wp_insert_post
. The second and third parameters are the priority and the arguments. We’ll get into that soon.
In our hooked function we simply define what we’d like to do. In this case send an email to ourselves. Whenever WordPress published a post it will look for all functions hooked into wp_insert_post
and run them all.
So what have we achieved here? We’ve added functionality to a core part of WordPress Themes without actually modifying the core files. All we needed was one extra line of code. This is very important! All you need to do is write a function that does what you need, then you hook it into a specific part of WordPress Themes.
Hooks, Actions, Filters, Hooked Function and Tags
There is some confusion about these terms above because sometimes they are used interchangeably. I myself mis-speak sometimes, so let’s get things straight just in case I mess up further down!
Hook is an umbrella term for actions and filters. Actions allow you to add your own functionality next to existing functionality. Filters allow you to modify existing functionality. Sometimes the word tag is used to refer to the string that indicates where you are adding your hook. A hooked function is a function you create that adds or modifies functionality.
The Anatomy of a Hook
When we use hooks we generally rely on two functions: add_action()
and add_filter()
. Both these function take four parameters:
- The first parameter is the tag. This is the bit that tells WordPress where to hook your function, when it should be executed.
- The second parameter should be the name of the hooked function.
- The third parameter is the priority of the hook. This determines the order in which it is executed if multiple functions are hooked into the same tag.
- The fourth parameter defines the number of parameters passed to this function. By default this is 1, but some tags (like the previous
wp_insert_post
) can have more.
Tag
The first parameter of both functions is a simple string that states when the hooked function is executed. There are many, many tags you can use. There are action tags that run when posts are deleted, categories are created, a user is logged in and more. Filter tags are run on messages for custom taxonomies, post title displays, dates and more. The Hooks Reference is a good place to start, but sometimes a Google search can be more effective!
Hooked Function
Again, a simple string, which should be the exact name of the function. Since this will be a function name it should contain letters, numbers and underscores only.
Take extra care to make this function unique. If you name a function “send_email” it may clash with someone else’s function of the same name. It is common practice to prefix function names, something like: my_send_email, or wpmudev_send_email.
I also advise making your function names readable. You could name your function sae()
, short for “send author email” but this is extremely bad practice as no one else will know what this means, and you will probably forget in a few months as well.
Hook Priority
The third function defines the priority of a hook. The lower the priority the sooner the function gets executed. In some cases the order is not important but in some cases it is essential. If you want to send a post publication email to yourself and the author, the order doesn’t much matter. You can use the default of 10 for both:
1 | add_action( 'wp_insert_post' , 'send_author_email' , 10, 3 ); add_action( 'wp_insert_post' , 'send_admin_email' , 10, 3 ); |
However, if you would like to update some statistics whenever a post is updated and then notify the author the order suddenly becomes important:
1 | add_action( 'save_post' , 'send_admin_email' , 10, 3 ); add_action( 'save_post' , 'update_post_stats' , 5, 3 ); |
As you can see, the order in which the add_action()
calls are places is not important. The second call has a lower priority, therefore it will be executed first.
Parameters
The fourth parameter sets the number of parameters passed to our hooked function. This is something you will need to hunt down as it depends on what is in the source code.
If you go back to see how we changed the login errors you’ll notice the following function: apply_filters( 'login_errors', $errors )
. The first parameter is the tag, the rest are arguments. There is only one here, so one argument is passed to our hooked function.
In case of wp_insert_post()
you can see the following in the core files: do_action( 'wp_insert_post', $post->ID, $post, true );
. The first parameter is the tag, the rest are arguments, so the value here is 3.
Conclusion
In this article we looked at the basics of hooks. We learned the difference between actions and filters and how to use them to effectively change the way WordPress works. The more you use hooks the more you’ll love them and appreciate the power and flexibility they offer.