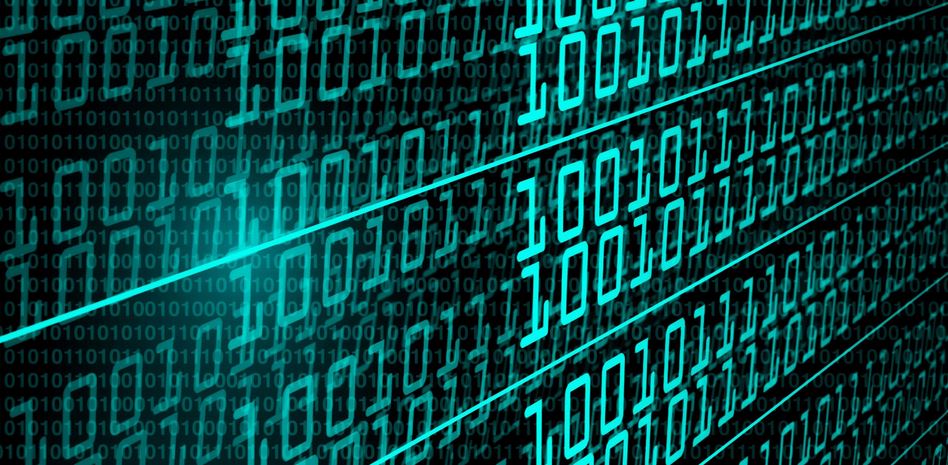
9 Simple Codes Enhancements for WordPress Themes Tweaks
The built-in settings and plugins just scratch the surface of what’s possible. Adding the snippets below to your wordpress themes/child themes or plugin, you can achieve some granular modifications.
1.Change Your Excerpt’s Length
Would you like to use an excerpt but you find the 55 word default a bit on the short side? You can modify it to your heart’s content using the excerpt_length
filter. It’s easy, the following will double the length of the excerpt to 110 words:
add_filter( 'excerpt_length', 'my_excerpt_length' ); function my_excerpt_length( $length ) { return 110; }
2.Redirect Single Search Result
When a user searches your website and there is only one result the user is taken straight to the single post, no need to click again in the search results.
This improves user experience, which leads to all sorts of benefits, including better statistics.
add_action( 'template_redirect', 'single_result' ); function single_result() { if ( is_search( )) { global $wp_query; if ( $wp_query->post_count == 1 ) { wp_redirect( get_permalink( $wp_query->posts['0']->ID ) ); } } }
3.Pretty Links
If you link to various sources frequently you could create a special link formatting to make them easily visible. For example: You could output the your site icon each time you link to an article.
When creating the link in the back-end make sure to add a class, something like prettylink-mageewp. You can then add a snippet like this to your stylesheet:
a.prettylink-mageewp::before { content: ''; margin-right:2px; position:relative; top:2px; width: 16px; height:16px; background: url('https://mysite.com/wp-content/uploads/2015/03/04/wordpressthemes.ico'); display:inline-block; }
4.Auto-Link Text
While on the subject of links, we can also create links from specific text. For example, whenever you mention WordPress you can convert the word to a link to the WordPress website. All you need to do is hook into the_content to modify the content of the post.
function auto_link_text( $content ){ $replacements = array( 'WordPress' => '<a href="https://wordpress.org">WordPress</a>' ); $content = str_replace( array_keys( $replacements ), $replacements, $content ); return $content; } add_filter( 'the_content', 'auto_link_text' );
This snippet replaces strings with links within the post content. If you’d like to do this in the excerpts as well, add another filter which hooks into the_excerpt.
Beware when using this method as it will convert text which is already contained in a link. If you occasionally link to WordPress anyway you will end up with broken HTML.
You could use some advanced regular expressions to make this happen but I would suggest creating links whenever you need one, only use this snippet as an interim solution.
5.Insert Content Between Paragraphs
In some scenarios you may want to insert your featured image after the first paragraph, or perhaps an ad after the second paragraph. This can be done by hooking into the_content and using some regular expression magic.
add_filter( 'the_content', 'my_content_insertion' ); function my_content_insertion( $content ) { preg_match_all('/<\/p>/', $content,$matches, PREG_OFFSET_CAPTURE); $insertion_point = $matches[0][1][1]; $content_before = substr( $content, 0, $insertion_point + 4 ); $content_after = substr( $content, $insertion_point + 5 ); $content_to_insert = get_the_post_thumbnail( $post->ID, 'post-single' ); return $content_before . $content_to_insert . $content_after; }
The key here is the $matches array. Increment the second number to add content after later paragraphs. $matches[0][3][1] will add your content after the fourth paragraph for example.
6.Redact Sensitive Content
If you have some authors prone to swearing but you don’t want to dictate their style you can create a neat little redactor by filtering the post content. You can enable this filter for guests only to make sure your logged in users see everything.
add_filter( 'the_content', 'my_redaction' ); function my_redaction( $content ) { $search = array( 'frak' => '<span class="redacted">karf</span>', ); $content = str_replace( array_keys( $search ), $search, $content ); return $content; }
We look for instances of frak and replace it with <span class="redacted">karf</span>
. We can then style the .redacted
class however we like, making it look like an actual redaction. I also jumbled up the letters because the word would otherwise still be visible in the source code, or if highlighted.
If you want to enable this for guest users only wrap all the code within the function in if ( !is_user_logged_in() )
.
7.Add Old Post Notices Automatically
Some websites write about rapidly growing and changing industries so posts from a year ago may well be outdated. In these cases you may want to add an outdated notice without having to go to each post and adding the shortcode. This can be done by hooking into the_content
yet again.
add_filter( 'the_content', 'outdated_notice' ); function outdated_notice( $content ) { $notice = "<p class='notice'>This content is more than a year old, it may be outdated!</p>"; $post_time = get_the_time( 'U' ); $current_time = current_time( 'timestamp' ); if( $current_time - $post_time >= 31556926 ) { $content = $notice . $content; } return $content; }
You’ll need to style this yourself, but with just three rules added it already looks nice in Twenty Fifteen.
8.Featured Image
Adds a featured image set from the single post Edit screen which displays before the content on single posts only.
add_filter( 'the_content', 'featured_image_before_content' ); function featured_image_before_content( $content ) { if ( is_singular('post') && has_post_thumbnail()) { $thumbnail = get_the_post_thumbnail(); $content = $thumbnail . $content; } return $content; }
9.Create Your Own Shortcodes
Shortcodes are a cinch to make and could make your life so much easier. You could use them to create the iconed links more easily, or to add more elaborate content with minimal effort.
Here are two examples:
add_shortcode( 'iconlink', 'iconlink' ); function iconlink( $atts ) { $atts = shortcode_atts( array( 'url' => false, 'text' => 'link' ), $atts ); if( empty( $atts['url'] ) ) { return; } $url = parse_url( $atts['url'] ); $domain = str_replace( '.', '-', $url['host'] ); return '<a href="' . $atts['url'] . '" class="' . $domain . '">' . $atts['text'] . '</a>'; } add_shortcode( 'update_notice', 'update_notice' ); function update_notice( $atts ) { $atts = shortcode_atts( array( 'url' => false, ), $atts ); if( empty( $atts['url'] ) ) { return; } return 'This post is a bit outdated so we have updated the contents with new developments and new methods. Please read <a href="' . $atts['url'] . '">the new version</a> for more information'; }
The first shortcode – iconlink – creates a link with a class which we can style with CSS, just like we did before. Here the class is generated automatically from the URL.
The second shortcode is a way to tell users to look at a new version of the post without having to type the notice each and every time. This is essentially a snippet, you just type ‘[update_notice url=”https://newcontent.com”]’ into the editor and you have your notice.
Conclusion
You can accomplish a lot with built-in settings and plugins and if you want complete control, code is your friend. Want to learn more? Please read below.