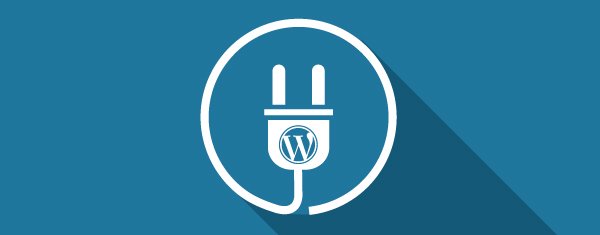
Basis for WordPress Themes Plugin Development
A plugin is a safe way to try out new things and the way to go if you need to implement cross-theme functions. This article will walk you through creating a simple plugin to have a look at some basic concepts about wordpress themes plugin.
A Simple Plugin Project
In this article we’re going to create a plugin that integrates WordPress and Facebook Open Graph. Open Graph tags are special HTML tags, which give Facebook the information it needs to share your page and ensure it looks great.
Here’s a set of example tags, which could be used on the page you are reading right now:
You can read all about Facebook Open Graph and Facebook Content Sharing Best Practices on the Facebook site.
For this project, we need to make sure that whenever a single blog article is shown, Open Graph tags are added to the header of our website. Site heads consists mostly of metadata and other hidden bits of information, and are added between the <head> and </head> tags in an HTML document.
The next part of this article will focus on getting this done. After this example, I’ll venture more deeply into plugin development territory.
Creating a New Plugin
The first thing you need to do is create a folder to store your plugin. Go to the wp-content/plugins/
directory in your WordPress installation and create a folder called my-facebook-tags
. Keep in mind that whatever you name your plugin’s folder will be your plugin’s slug.
A plugin slug should be unique throughout the WordPress Plugin Repository if you want to upload it and make it publicly available. What this means is that no other plugin created by anyone else should have this slug. You can search for existing plugin slugs easily, just use Google!
Keep in mind that the plugin’s name is not necessarily the same as its slug. Take a look at the iThemes Security plugin. The last bit of the URL is the slug: better-wp-security. The name of the plugin, however, is iThemes Security.
If you’re just making a plugin for yourself it is still important to make sure slugs don’t clash. During the lifetime of your website you will probably use a number of plugins and you don’t want one to accidentally clash with yours and cause problems on your site because of a naming conflict.
Now that you have your my-facebook-tags
folder, create a new file inside and name itmy-facebook-tags.php
. This will be your main plugin file and its name should be the same as your plugin’s slug, with the PHP extension tacked on.
Open your plugin’s main file and paste in the following code:
<?php /** * Plugin Name: My Facebook Tags * Plugin URI: https://your.com * Description: This plugin adds some Facebook Open Graph tags to our single posts. * Version: 1.0.0 * Author: Daniel Pataki * Author URI: https://your.com * License: GPL2 */
This code is a PHP comment, which won’t be visible directly in the WordPress admin. WordPress does use the data within it to output the plugin’s name and some other data in the Plugins section of the backend. Please modify the plugin author and other strings as you see fit.
Once you’ve saved this file, congratulation are in order because you’re just create your first plugin! It does absolutely nothing, of course, but it should be available in the plugins section and you should be able to activate it – go ahead and do that now.
How Plugins Work
Let’s pause for a moment to look at how plugins work before we continue with our Facebook Open Graph project.
Plugins provide functionality with hooks, therefore understanding how they work is crucial. Let’s look at a real world analogue for hooks. You know those little diaries where the first sentence says: I am the diary of _________. The empty line is where you put your actual name.
The company could of course go through all the names and create prints of each one but it would not be economical and a lot of people would be left out. Also, what if you want to put “The Master Of The Galaxy” instead of your own name?
That blank line is a hook. Instead of being specifically printed for a person it prompts the user to add his/her own name. Hooks work something like this in WordPress, let’s look at an example.
Themes are required to add the following function to the header file: wp_head()
. Within this function is a bit of code where WordPress says: If a plugin wants to put some code here they may do so. The wp_head
hook allows us to output something in the head section of the page, which is exactly what we need. Let’s test this”
add_action( 'wp_head', 'my_facebook_tags' ); function my_facebook_tags() { echo 'I am in the head section'; }
The first line of the snippet above tells WordPress that we would like to attach some functionality to the wp_head
hook using the my_facebook_tags()
function.
The second line of code creates that function and the third line echoes a simple string.
This should now be visible at the top of any theme you activate, as long as it defines that wp_head()
function (defining it is a requirement). We’ll remove that echoed string soon since you should never display text in the head section.
For the sake of correctness let me mention two things. There are two types of hooks: actions and filters. In the case above we used an action which is obvious because we used the add_action()
function. Actions run whenever WordPress detects the hook that calls them.
Filters are similar but they modify a bit of data which WordPress uses. A good example is the logout message that is shown. Instead of performing an action whenever a logout message is shown, a filter allows you to modify the logout message itself. We will not go into hooks in detail here.
Completing Our Plugin
Based on the description above it’s pretty clear we need to add our Facebook meta tags using the wp_head
hook.
Here’s the rest of the code needed for our plugin, followed by an explanation:
add_action( 'wp_head', 'my_facebook_tags' ); function my_facebook_tags() { if( is_single() ) { ?> <meta property="og:title" content="<?php the_title() ?>" /> <meta property="og:site_name" content="<?php bloginfo( 'name' ) ?>" /> <meta property="og:url" content="<?php the_permalink() ?>" /> <meta property="og:description" content="<?php the_excerpt() ?>" /> <meta property="og:type" content="article" /> <?php if ( has_post_thumbnail() ) : $image = wp_get_attachment_image_src( get_post_thumbnail_id(), 'large' ); ?> <meta property="og:image" content="<?php echo $image[0]; ?>"/> <?php endif; ?> <?php } }
I’ve basically pasted our meta tags into the function as-is. The only things I needed to modify were the values to make sure they reflected the currently shown post. I used the is_single() conditional tag to make sure that the tags are only added when a single post is shown.
In order to use the title, excerpt, image, etc of the current post I used template tags. The only bit of trickery I used was to check if the post has a featured image before displaying the Facebook tag for it.
With this single function in place we’ve created something quite useful. All of the posts on your website should now have Facebook-friendly tags. You can make sure they’re set up properly using the Open Graph Debugger.
And now our plugin is complete.
Related Articles
Translating WordPress Themes Plugins