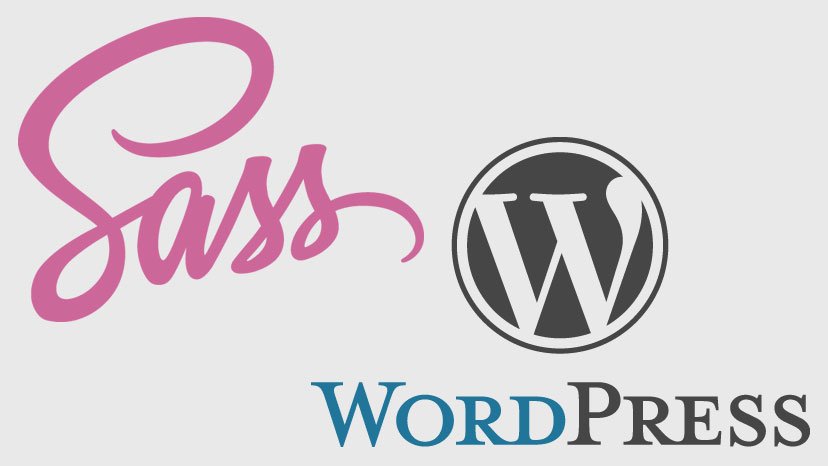
Using Sass in WordPress Themes
Why Do We Need Sass
Sass is a CSS preprocessor: a programming language that compiles to (spits out) CSS stylesheets. Like any CSS preprocessor, Sass exists to let you write styling rules in a manner that is:
- DRY: “don’t repeat yourself,” meaning less copy-pasting and blind repetition.
- Dynamic: capable of interpreting variables.
WHAT DOES NON-DRY CSS LOOK LIKE?
Let’s look at one example of “non-DRY CSS,” and why it might be an issue:
.nav { font-family: "Georgia", serif; } .nav .nav-element { display: inline-block; } .nav .nav-element .nav-dropdown { background: #eee; }
Pure CSS is flat: there’s no way to indicate structure, except inside an individual styling rule. In a highly structured stylesheet, this means a lot of repeating of “.nav
,” “.nav-element
,” and so forth.
Now imagine, what happens if we rename “.nav” to “.nav-menu“—or get rid of .nav altogether? We’re going to have to manually rewrite every styling rule we wrote that depended on .nav. In other words: The code repeats itself, so one change breaks it in multiple places.
CSS IS STATIC; WHAT’S WRONG WITH THAT?
Let’s look at an example:
.small-rounded-square { height: 16px; width: 16px; border: 1px #333 solid; border-radius: 1px; } .medium-rounded-square { height: 32px; width: 32px; border: 2px #333 solid; border-radius: 2px; } .large-rounded-square { height: 48px; width: 48px; border: 3px #333 solid; border-radius: 3px; } .xl-rounded-square { height: 64px; width: 64px; border: 4px #333 solid; border-radius: 4px; }
See the problem? These are all squares, meaning their width equals their height automatically. Moreover, they’ve all got a dark gray border equal to 1/16 of their width. And yet you have to write that all out by hand—giving us more non-DRY code that will be difficult to maintain and change.
How Sass Helps
Let’s look at the two code snippets above, done the Sass way:
.nav { font-family: "Georgia", serif; .nav-element { display: inline-block; .nav-dropdown { background: #eee; } } }
See? Sass has nesting!
Notice that this allows us to name each element only once. If we change (or even remove) .nav
in our HTML markup, we only have to change one thing in our Sass to reflect that change, instead of potentially dozens in vanilla CSS. The code above compiles to (spits out) exactly the CSS code in the first example—but you’ll only ever need to write and maintain the simpler version.
When and whether to nest your CSS rules is a subject of debate, but the point is that with Sass you can actually do it properly.
@mixin square($side: 16px) { width: $side; height: $side; border: $side/16 #333 solid; border-radius: $side/16; } .small-rounded-square { @include square; } .medium-rounded-square { @include square(32px); } .large-rounded-square { @include square(48px); } .xl-rounded-square { @include square(64px); }
Here, we define a mixin called “square” that captures the important shared properties of the squares we’re working with. Our mixin accepts a variable value—$side
, set to a default of 16px if nothing’s passed in)—just as a PHP or JavaScript function might.
Then, when we go to define our actual squares, we simply @include
square—like calling a function in PHP—giving it a custom value for $side
if we don’t want it to be 16px.
The huge advantage of this way of doing things is that you can standardize and centralize the properties of your square
elements. Want to get rid of the dark-gray borders across the site, or maybe give the squares a light gray background? You only have to go one place to do it: the square
mixin definition. All your square elements, of all sizes, will inherit the styles automagically.
PRETTY COOL, RIGHT?
Starting to see the promise of Sass? I’ll tell you seriously that I don’t know how I would have done the new WPShout theme (or another recent large project I completed a few months ago) without it. Once you’re able to start nesting elements, referring to variables (font-size: $base-font-size
), and dropping in repeatable bits of code, it’s just about impossible to consider going back.
How Sass and WordPress Interact
Working in a WordPress themes project is a bit different than working on, say, a greenfield web application powered by a JavaScript framework. The WordPress environment is set up in a pretty specific way, and there are only a few times when you’ve got a giant, blank stylesheet staring you in the face—which is where Sass really shines.
DRAWBACKS OF SASS
I’ll also mention three potential drawbacks of Sass, in order of importance:
- Sass means one more layer of abstraction preventing a beginner user from understanding what you’re doing. Compiled stylesheets are not necessarily as readable as hand-written ones—and can be completely opaque if you don’t think about the CSS your Sass is spitting out—and even readable CSS stylesheets don’t always encode the underlying logics that guided the original Sass programmer.
- Sass creates the possibility of versioning conflicts: future users of your software can make changes either to your CSS stylesheets or your Sass partials, and can potentially end up overwriting one another in the process.
- Writing Sass and getting it to compile is significantly more complicated, and marginally slower, than simply writing straight to a CSS file.
WHEN TO USE SASS AS A WORDPRESS DEVELOPER
So Sass isn’t cost-free. When to use it? Based on my experience with Sass and WordPress, I’d say I’d endorse the following very rough rules of thumb:
- You should definitely use Sass if you’re building a theme from scratch. It’ll save you dozens of hours, and the final product will be so much better.
- You should probably use Sass if you’re building a child theme. Really, it depends on how extensive the CSS modifications you’re making: if you’re going to turn a stock theme like Twentyfifteen into something totally different, Sass is a no-brainer; but for a couple of stray recolorings and font changes I’d say it can be overkill.
- I’m not sure you should use Sass if you’re building a plugin or modifying an existing theme. An existing theme is pretty much the tangle of wires and silly string that it is; adding Sass on top of everything might be a bit like dumping a brand-new file cabinet on top of a trash heap, on the theory that it’ll “organize everything.” As for plugins, the power of Sass makes sense in some cases (say, for a landing page plugin with a lot of design options), but may again be overkill for others.
Getting Started in Sass
There’s no way this post can teach you everything—or even very much—about how to use Sass. But here are some resources:
COMPILERS
The hardest part about Sass is getting it to compile. This usually requires someone who understands command-line Ruby, as well as a server that can run Ruby. That’s the kind of technical hurdle that often keeps me from even considering cool tech tools like Sass.
That’s why the biggest single Sass breakthrough for me has been finding Koala, a GUI-based desktop app that automatically compiles Sass to CSS for you. It’s dead-simple on Windows, and claims to work on Mac and Linux as well. The tutorials below also list a number of other compilers, which I expect work but haven’t tried.